Testing code is an integral part of any software development process, and React is no exception. When dealing with code that causes React updates to be wrapped, it’s crucial to understand the nuances to ensure optimal performance.
This article aims to shed light on this complex yet fascinating aspect of React coding. It’ll delve into the intricacies of testing code that triggers wrapped React updates, simplifying the concept for beginners, and providing in-depth insights for experienced developers.
When Testing, Code That Causes React State Updates Should Be Wrapped Into Act(…):
The React updates mechanism plays a pivotal role in any React application. During its lifecycle, a React component renders several times, and it’s these render cycles that are called updates. To have an optimized performance, understanding how to interact with and control these updates becomes essential.
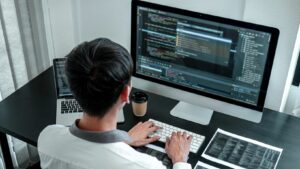
Categorically, React updates are driven by two events: when the component’s state changes and when the component’s props change. For instance, in the first event, toggling a light switch in a UI interface would signify a state change, enabling React to intervene and trigger a UI re-render.
Concurrently, the React engine governs whether a component needs re-rendering. It compares the component’s previous and next state or prop values using a method called shouldComponentUpdate()
. By default, React assumes that every state or prop change requires a re-render.
Yet, developers can override this default behavior by either utilizing shouldComponentUpdate()
in class components or React.memo()
in functional components. Both these methods direct React on when to skip unnecessary render cycles, consequently enhancing an application’s performance.
Key Challenges in Testing React Updates
Diving into testing React updates presents multiple hurdles. Digging through these, several challenges crop up consistently. Isolating updates, ensuring assertive stakeholder evaluations, and component lifecycle can pose prickly problems.
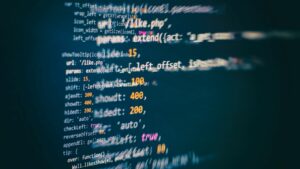
- Isolating updates ranks paramount among challenges. Updates in React cannot be cherry-picked for testing. All updates in a component render in a batch, making it challenging to isolate and test individual updates.
- Assertive stakeholder evaluations constitute another hurdle. Achieving an assertive evaluation of a component’s updated state often becomes troublesome. The confusion arises due to React’s async nature for updates, which can lead to inaccurate interpretations of a component’s status during tests.
- Component lifecycle challenges round out the list. Methods such as
componentDidUpdate()
, if not used with caution, can cause an endless loop of updates. For instance, if a setState function gets called incomponentDidUpdate()
, without a controlling condition, it’ll trigger an infinite loop of updates.
In all, these challenges, among others, stand tall in the path of effective React update testing. Understanding them aids in crafting comprehensive and effectual testing strategies.
Best Practices for Testing Wrapped Updates in React
Adopt the Jest framework, favored by React developers. Jest shines due to its automatic mocking, timers control, and asynchronous testing support.
Opt for shallow rendering during testing, a function provided by Enzyme. It permits the testing of components in isolation, benefitting from reduced complexity and increased speed. It isolates a single component, testing without deep rendering child components.
Count test coverage with tools like Istanbul. They generate comprehensive coverage reports that identify untested sections of code. With 70-80% of code coverage recommended, Istanbul ensures you’re on the right track.
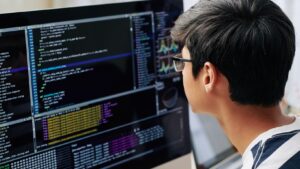
Depend on the Act() function in test scenarios. It helps encapsulate values that may alter over time due to state changes, marking them as important to the React reconciler.
Mock UI events through React Testing Library. For example, fireEvent, UserEvent, and screen render assist in carrying out UI events such as clicks and form inputs.
Provide input values using Test Renderer. It acts as a counterpart to browser’s DOM, facilitating the compilation of JSX to JavaScript and managing component trees for testing.
Use the cleanup function to simplify tests. Automatically executed after each test, it ensures component unmounting, preventing any test interference.
Employ callback functions within the wrapper. It’s useful in synchronizing state updates and the component rendering process, primarily when testing conditional rendering.
Embrace the use of the React Profiler API for performance testing. It captures timing information about each component rendered as part of “commit,” helping to identify slow components.
Practicing these steps, your React code testing becomes a more controlled, efficient, and accurate exercise, delivering high-quality deliverables every time.